Juggling complex data within a website can be a daunting task. Traditional posts and pages, while fundamental building blocks of WordPress, often fall short when managing intricate information. Imagine trying to showcase a vast array of hair extensions, colour techniques, and styling services offered by a luxury hair salon – the limitations of standard post formats become readily apparent. This is where custom post types (CPTs) come into play.
The Power of CPTs
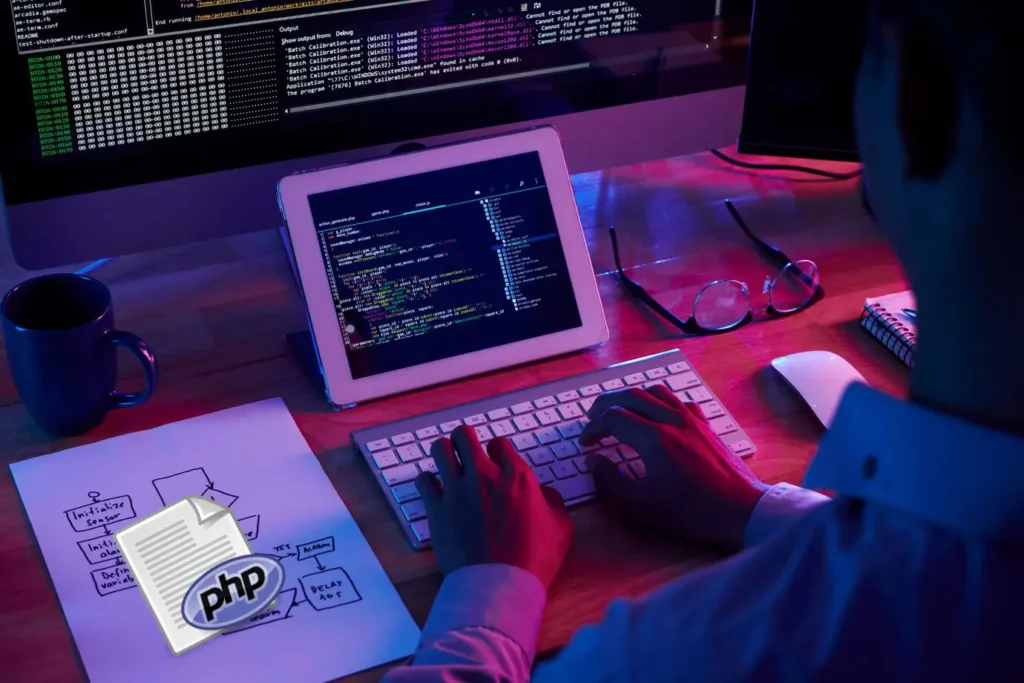
Custom post types are a game-changer for websites that require a more structured approach to data management. They essentially allow you to create bespoke content types tailored to your specific needs. This translates to a website that’s organised, user-friendly, and future-proof. Think of it as building a dedicated filing system for your content, as opposed to throwing everything into a single, overflowing folder.
Let’s take Amaraa Hair Salon, Perth’s leading luxury hair salon, for example. They specialise in a wide range of services, from hand-tied weft hair extensions to keratin smoothing treatments. Using traditional posts would force them to cram all this information into generic post formats, making it difficult for both website visitors and administrators to navigate.
However, by utilising CPTs, Amaraa Hair could create dedicated content types for each service category – “Hair Extensions,” “Keratin Treatments,” “Hair Colour,” and “Hair Styling.” Within each CPT, they could then define specific fields to capture relevant details. For hair extensions, this might include hair type, length options, and colour choices. For keratin treatments, specific treatment types and expected results could be captured. This level of granular control empowers Amaraa Hair to present their services in a clear, organised manner, ultimately enhancing the user experience and showcasing their expertise.
Building Your Scalable Structure
Now that we understand the power of CPTs, let’s delve into the practicalities of creating them. Here, we’ll provide code snippets to guide you through the process.
A. Registering Custom Post Types
The first step is registering your custom post type with WordPress. This is achieved by adding code to your theme’s functions.php file. Here’s an example:
PHP
function create_hair_service_cpt() {
$labels = array(
'name' => __( 'Hair Services' ),
'singular_name' => __( 'Hair Service' ),
'menu_name' => __( 'Hair Services' ),
'parent_item_colon' => __( 'Parent Hair Service:' ),
'all_items' => __( 'All Hair Services' ),
'view_item' => __( 'View Hair Service' ),
'add_new_item' => __( 'Add New Hair Service' ),
'edit_item' => __( 'Edit Hair Service' ),
'update_item' => __( 'Update Hair Service' ),
'search_items' => __( 'Search Hair Services' ),
'not_found' => __( 'No Hair Services found' ),
'not_found_in_trash' => __( 'No Hair Services found in Trash' ),
);
$args = array(
'labels' => $labels,
'public' => true,
'has_archive' => true,
'rewrite' => array( 'slug' => 'hair-services' ),
'menu_icon' => 'dashicons-scissors', // Choose an appropriate icon
'supports' => array( 'title', 'editor', 'thumbnail' ),
);
register_post_type( 'hair_service', $args );
}
add_action( 'init', 'create_hair_service_cpt' );
This code defines a custom post type called “Hair Service.” You can customise the labels to match your specific terminology (e.g., “Treatment” instead of “Service”). The supports
argument specifies what features are enabled for this CPT, such as titles, an editor, and featured images.
B. Customising Content with Fields
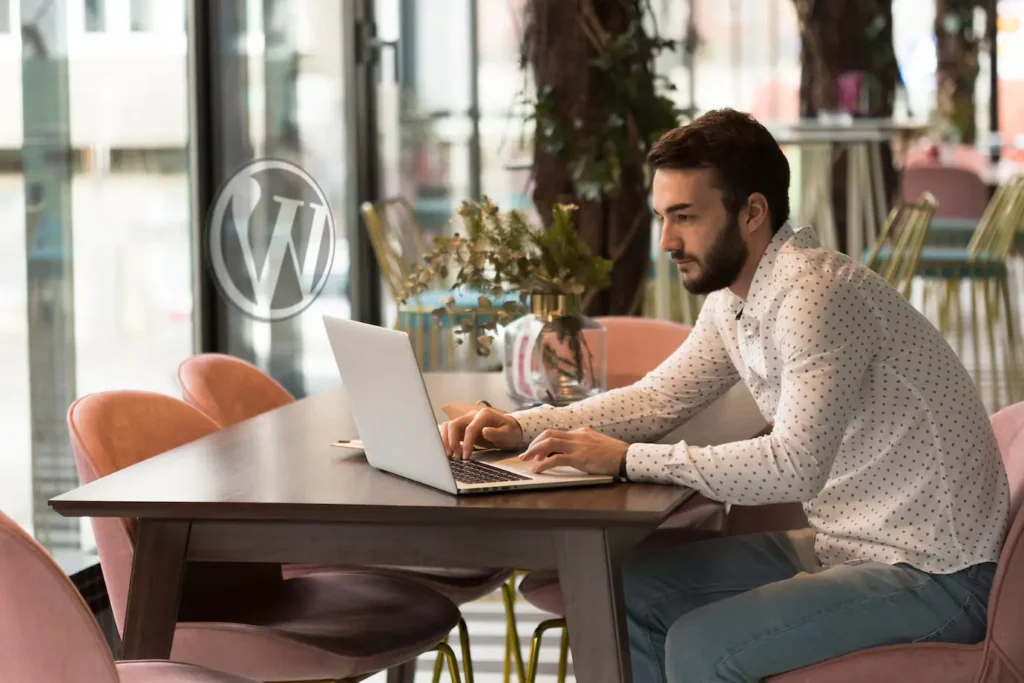
While creating a custom post type offers a structure, it’s the custom fields that truly bring it to life. These fields allow you to capture the specific data points relevant to your content. Here’s how to add custom fields using the Advanced Custom Fields plugin (a popular choice):
Let’s say you want to create a custom field for “Hair Type” within your “Hair Extension” CPT. You would use the Advanced Custom Fields plugin interface to create a new field group named “Hair Extension Details.” Within this group, you would create a new field of type
Within this group, you would create a new field of type “Select” and name it “Hair Type.” You can then define the various hair type options (e.g., Remy hair, Virgin hair, Synthetic hair) as choices within the field. Additionally, you can create separate fields for “Length” (using a dropdown menu) and “Colour” (using a colour picker or a text field with colour codes).
Here’s a breakdown of some common field types and their uses:
- Text: Ideal for capturing short pieces of information like service durations or product descriptions.
- Textarea: Perfect for longer descriptions or detailed instructions.
- Select: Useful for offering pre-defined options, such as hair types, treatment durations, or colour choices.
- Checkbox: Allows for selecting multiple options, like highlighting various benefits of a service.
- Radio Button: Useful for single-choice selections, like choosing a specific stylist or consultation type.
- Image: Enables uploading images associated with the content, such as showcasing different hair extension styles.
- Gallery: Allows for adding multiple images to showcase a service or product in detail.
By utilising these different field types, you can create a comprehensive data structure for your custom post type. This empowers you to capture all the information crucial for effectively presenting your content.
C. Putting it All Together
Here’s an example combining the previous code snippets to create a custom post type “Hair Extension” with relevant custom fields:
PHP
function create_hair_extension_cpt() {
$labels = array(
'name' => __( 'Hair Extensions' ),
'singular_name' => __( 'Hair Extension' ),
// ... (other label definitions)
);
$args = array(
'labels' => $labels,
'public' => true,
'has_archive' => true,
'rewrite' => array( 'slug' => 'hair-extensions' ),
'menu_icon' => 'dashicons-extensions',
'supports' => array( 'title', 'editor', 'thumbnail' ),
);
register_post_type( 'hair_extension', $args );
}
add_action( 'init', 'create_hair_extension_cpt' );
// Custom Fields using Advanced Custom Fields plugin (replace with your actual plugin code)
if ( function_exists('acf_add_local_field_group') ):
acf_add_local_field_group(array(
'location' => array(
array(
array(
'param' => 'post_type',
'operator' => '==',
'value' => 'hair_extension',
),
),
),
'fields' => array(
array(
'label' => 'Hair Type',
'type' => 'select',
'name' => 'hair_type',
'choices' => array(
'remy_hair' => 'Remy Hair',
'virgin_hair' => 'Virgin Hair',
'synthetic_hair' => 'Synthetic Hair',
),
),
array(
'label' => 'Length',
'type' => 'select',
'name' => 'hair_length',
'choices' => array(
'14_inches' => '14 Inches',
'16_inches' => '16 Inches',
'18_inches' => '18 Inches',
'20_inches' => '20 Inches',
),
),
array(
'label' => 'Colour',
'type' => 'text',
'name' => 'hair_colour',
'placeholder' => '#ColorCode or Descriptive Colour Name',
),
// Add more fields as needed (e.g., price, additional details)
),
'title' => 'Hair Extension Details',
));
endif;
This code creates a custom post type “Hair Extension” with basic functionality and allows you to define hair type, length, and colour using custom fields.
Advanced Applications of CPTs (Optional)
While the core functionalities of CPTs are powerful on their own, there are ways to extend their capabilities for even more complex data management:
- Creating Custom Relationships Between CPTs: Imagine Amaraa Hair wants to showcase stylists who specialise in specific hair extension techniques. By creating a custom post type “Stylist” and establishing a relationship with “Hair Extension,” you can connect stylists with their areas of expertise. This allows for more intricate data organisation and enriches the user experience.
- Utilizing Custom Taxonomies for Further Data Organization: Taxonomies are essentially classification systems within WordPress. You can create custom taxonomies to further categorize your content within a CPT. For Amaraa Hair, you might create a custom taxonomy “Hair Type” with categories like “Straight,” “Wavy,” and “Curly” to further refine their hair extension offerings.
- Integrating CPTs with Custom Plugins: For highly specific needs, consider developing custom plugins that interact with your CPTs. This allows for functionalities beyond what core WordPress offers. For instance, a custom plugin could integrate with a booking system, allowing clients to schedule appointments for specific hair extension services directly through the Amaraa Hair website.
Conclusion
Custom post types empower you to move beyond the limitations of traditional posts and pages. They offer a structured approach to data management, making your website scalable and user-friendly. By leveraging CPTs and their customization options, you can create a website that effectively represents your brand or service, just like Amaraa Hair Salon can now showcase their extensive range of hair services in a clear and organized manner.
Bonus: Addressing Common Challenges with CPTs
- Managing Large Datasets: As your data grows, consider implementing pagination or filtering mechanisms to ensure smooth website performance. Plugins can help streamline data management for large CPTs.
- Optimizing for Performance: Ensure efficient queries and avoid unnecessary database calls when working with CPTs. Caching plugins can also help improve website speed.
Further Resources:
- WordPress Documentation on Custom Post Types: https://developer.wordpress.org/plugins/post-types/
- Advanced Custom Fields Plugin: https://www.advancedcustomfields.com/
Remember, this is just the beginning! As you explore the world of CPTs, you’ll discover even more ways to tailor your website to your specific needs and create a truly exceptional user experience.