Building Custom Taxonomies and Relationships in WordPress (with Code Examples)
The way you organise your content on a website is crucial. A well-structured website is easier for visitors to navigate, improves user experience, and can even boost your search engine optimisation (SEO). While WordPress offers built-in taxonomies for categorising content, these can become limiting for complex websites with diverse content types. This is where custom taxonomies and relationships come in.
When Basic Taxonomies Fall Short
Imagine you’re building a website for a travel blog. You might want to use categories like “destinations” and “travel tips.” However, these basic taxonomies wouldn’t allow you to categorise content by specific regions, trip types (solo travel, family holidays), or travel styles (luxury, budget). This lack of flexibility can lead to navigation issues for users who might struggle to find the content they’re looking for. Additionally, with poorly organised content, search engines might have difficulty understanding the relationships between different pieces of content, potentially impacting your SEO ranking.
The Power of Custom Taxonomies
Custom taxonomies essentially act as new classification systems for your content, allowing you to categorise it in a way that best suits your specific needs. Unlike default categories and tags, custom taxonomies can be hierarchical (like a parent-child structure) or non-hierarchical (allowing for more flexibility). You can create multiple custom taxonomies and even establish relationships between them for even more sophisticated content organisation.
For instance, on your travel blog, you could create custom taxonomies for “regions” (hierarchical, with subcategories for specific countries), “trip types” (non-hierarchical), and “travel styles” (non-hierarchical). This allows you to categorise a blog post about backpacking in Southeast Asia as “Southeast Asia” (region), “Backpacking” (trip type), and “Budget” (travel style).
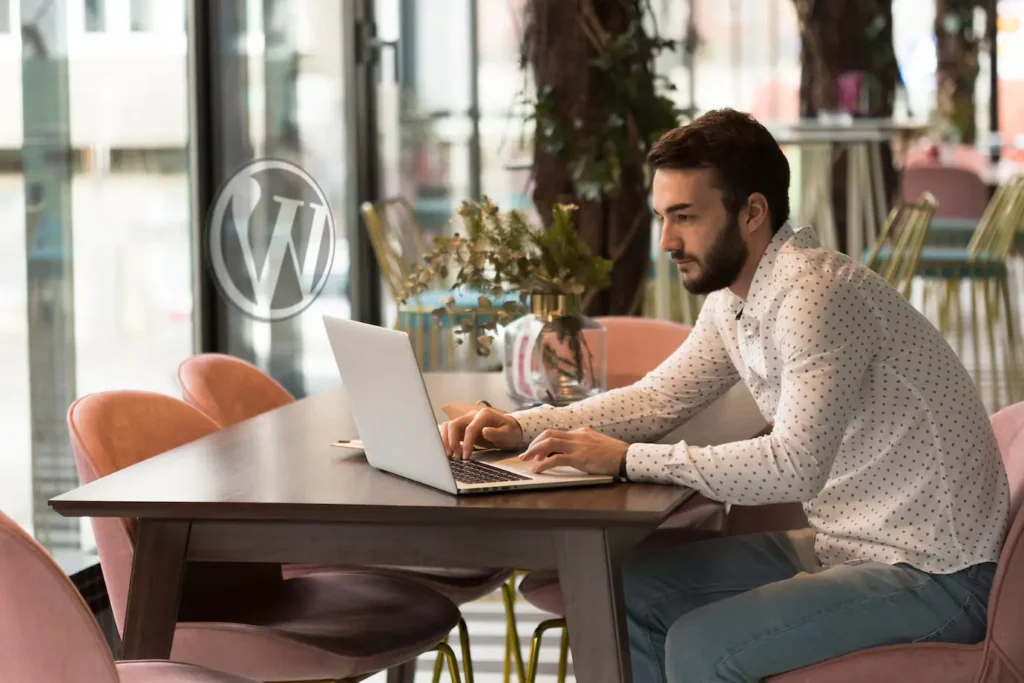
Getting Started: Using Plugins for Custom Taxonomies (For Beginners & Intermediates)
If you’re a beginner or intermediate WordPress user who isn’t comfortable with code, fear not! Several user-friendly plugins can help you create custom taxonomies without writing a single line of code. Popular options include “Custom Post Type UI” and “Taxopress.” These plugins offer a user-friendly interface with drag-and-drop functionality, allowing you to easily define custom taxonomies, configure labels, and assign them to specific post types.
Using plugins simplifies the process and allows you to experiment with custom taxonomies without needing advanced coding knowledge. However, if you’re comfortable with code or want more granular control over your custom taxonomies, then diving into code is the way to go.
Building Custom Taxonomies with Code (Step-by-Step)
Here’s a step-by-step guide on creating custom taxonomies in WordPress using code snippets:
1. Register Your Custom Taxonomy:
The first step involves registering your custom taxonomy with WordPress. You can achieve this by adding a function to your theme’s functions.php file. Here’s an example:
PHP
function create_custom_taxonomy() {
$labels = array(
'name' => __( 'Regions' ),
'singular_name' => __( 'Region' ),
'plural_name' => __( 'Regions' ),
'menu_name' => __( 'Regions' ),
// ... (other label definitions)
);
$args = array(
'hierarchical' => true, // Set to 'false' for non-hierarchical taxonomies
'labels' => $labels,
'public' => true,
'show_ui' => true,
// ... (other argument definitions)
);
register_taxonomy( 'custom_region', array( 'post', 'page' ), $args ); // Replace 'custom_region' with your desired slug
}
add_action( 'init', 'create_custom_taxonomy' );
This code snippet defines a custom taxonomy called “custom_region” with specific labels and arguments. You can customise the labels to match your desired terminology and adjust the arguments based on your needs (e.g., setting “hierarchical” to false for non-hierarchical taxonomies).
2. Assigning Custom Taxonomies to Post Types:
Once you’ve registered your custom taxonomy, you need to associate it with specific post types. Here’s how:
PHP
function add_taxonomy_to_post_types() {
register_taxonomy_for_object_type( 'custom_region', 'post' ); // Replace 'custom_region' with your slug and 'post' with your desired post type(s)
}
add_action( 'init', 'add_taxonomy_to_post_types' );
Now that you can create custom taxonomies, let’s explore how to establish relationships between them for even more powerful content organisation. There are two main types of relationships:
- Hierarchical: Similar to categories, where a parent term can have child terms. This is useful for situations where one taxonomy inherently relates to another in a parent-child structure.
- Non-Hierarchical (Many-to-Many): This allows a single piece of content to be associated with multiple terms from different taxonomies. This is ideal for scenarios where content can be categorised in various ways without a strict hierarchy.
Here’s how to implement these relationships using code:
1. Hierarchical Relationships:
WordPress doesn’t natively support creating hierarchical relationships between custom taxonomies. However, you can achieve this by utilising a custom post type as an intermediary. Here’s a simplified example:
PHP
// Register a custom post type for relationships
function create_relationship_post_type() {
register_post_type( 'taxonomy_relationship', array(
'public' => false,
'show_ui' => false,
) );
}
add_action( 'init', 'create_relationship_post_type' );
// Function to establish a hierarchical relationship between terms
function create_hierarchical_relationship( $parent_term_id, $child_term_id ) {
$args = array(
'post_type' => 'taxonomy_relationship',
'post_title' => wp_generate_uuid(), // Unique identifier
'meta_input' => array(
'parent_term' => $parent_term_id,
'child_term' => $child_term_id,
),
);
wp_insert_post( $args );
}
This code creates a hidden custom post type called “taxonomy_relationship” to store the relationship data. The create_hierarchical_relationship
function takes the IDs of the parent and child terms and creates a new post with metadata linking them.
2. Non-Hierarchical (Many-to-Many) Relationships:
For many-to-many relationships, you can leverage the built-in WordPress function wp_set_object_terms
. This function allows you to associate a specific post type with multiple terms from different custom taxonomies.
PHP
function assign_multiple_terms( $post_id, $term_ids, $taxonomy ) {
wp_set_object_terms( $post_id, $term_ids, $taxonomy );
}
// Example usage: associating a post with terms from two custom taxonomies
$post_id = 123; // Replace with your actual post ID
$region_terms = array( 5, 7 ); // Replace with your term IDs
$trip_type_terms = array( 2 ); // Replace with your term IDs
assign_multiple_terms( $post_id, $region_terms, 'custom_region' );
assign_multiple_terms( $post_id, $trip_type_terms, 'custom_trip_type' );
This code snippet defines a function assign_multiple_terms
that takes the post ID, an array of term IDs, and the custom taxonomy slug. You can then call this function to associate a post with terms from different taxonomies.
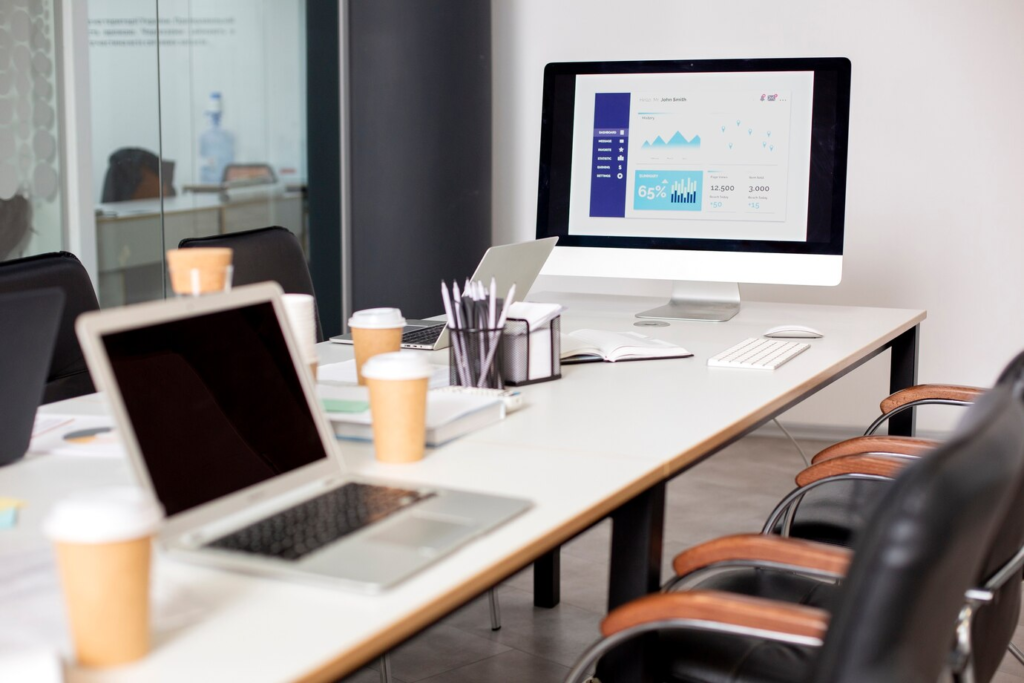
Advanced Use Cases and Customization (for Developers)
Custom taxonomies offer immense flexibility for complex content structures. Here are some advanced use cases:
- Integrating with Custom Post Types: Combine custom taxonomies with custom post types for even more granular content management. For example, create a custom post type for “Recipes” and use custom taxonomies for “Cuisine,” “Dietary Restrictions,” and “Cooking Difficulty.”
- Custom Fields and Conditional Logic: Integrate custom fields with your taxonomies to capture additional information and use conditional logic to display content based on specific terms.
For developers, custom taxonomies open doors to further customization using hooks and filters. You can modify how taxonomies are displayed, restrict access based on user roles, and even create custom meta boxes for managing taxonomy data.
Conclusion
Custom taxonomies and relationships empower you to organize your WordPress content in a way that best suits your specific needs. They provide greater flexibility, improve user experience, and can even enhance your SEO efforts. Whether you’re using plugins or diving into code, custom taxonomies can truly supercharge your content structure.
For further exploration, consider these resources:
- WordPress Codex: https://developer.wordpress.org/reference/functions/register_taxonomy/
- Custom Post Type UI Plugin: https://wordpress.org/plugins/custom-post-type-ui/
But WordPress’s capabilities extend far beyond content organization. In today’s digital landscape, websites often require functionality beyond their core features. This is where integrating third-party APIs comes in. By leveraging external services through APIs, you can significantly enhance the user experience.