Bridging the Gap: Seamless Third-Party API Integration in WordPress with cURL (Code Snippets Included)
While WordPress offers built-in functionalities, they can be limited when dealing with complex API interactions. This is where cURL comes in. cURL is a powerful library allowing developers to make HTTP requests and retrieve data from various APIs. By harnessing cURL’s capabilities, you can seamlessly integrate external services and unlock new possibilities for your WordPress projects.
This guide delves into the world of cURL for WordPress developers. We’ll explore the basics of cURL, provide a step-by-step approach to API integration, showcase practical code snippets, and discuss security considerations. Buckle up and get ready to bridge the gap between your WordPress website and the vast ecosystem of third-party APIs.
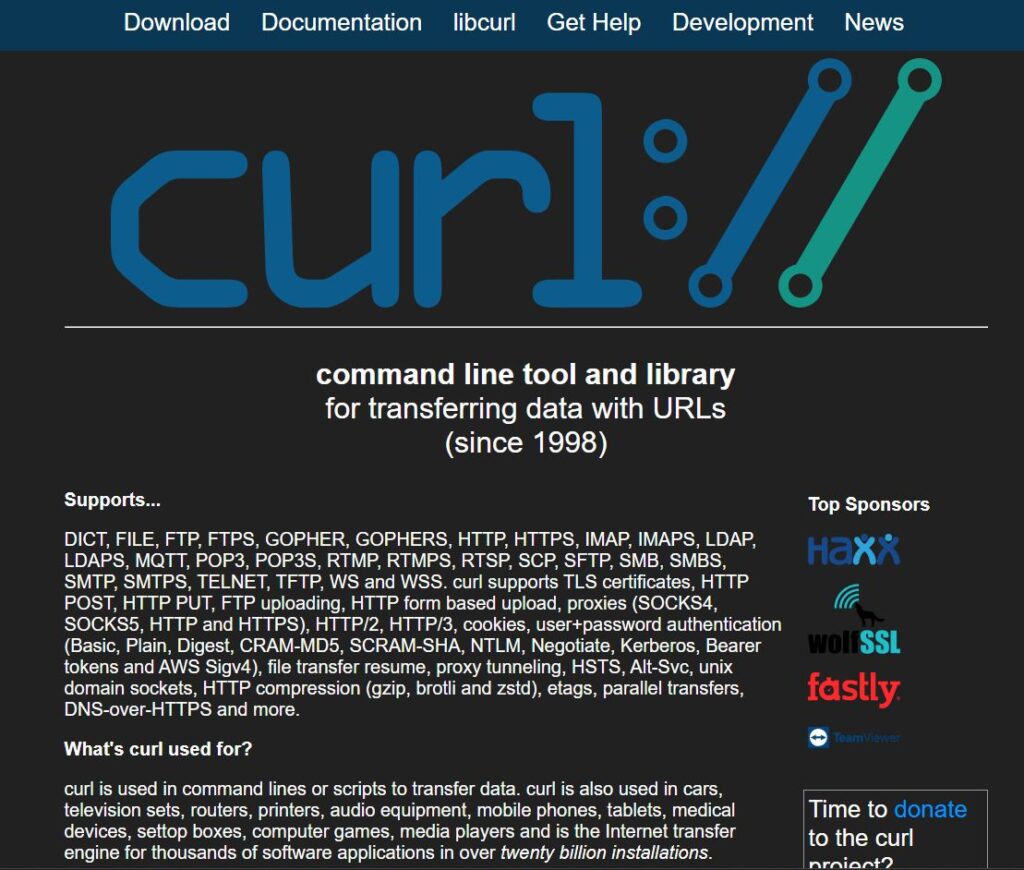
Understanding cURL for WordPress Developers
cURL is a command-line tool and library used for transferring data over various protocols like HTTP, FTP, and HTTPS. It offers granular control over HTTP requests, allowing developers to specify headers, authentication methods, request types (GET, POST, PUT, etc.), and data formats like JSON or XML. This flexibility makes cURL a versatile tool for interacting with diverse APIs.
Integrating APIs often involves fetching data from an external server. cURL facilitates this process by initiating a request to a specific API endpoint (URL) and retrieving the response data. This retrieved data can then be formatted and utilised within your WordPress website.
Step-by-Step Guide to API Integration with cURL
Now, let’s dive deeper and explore the practical steps involved in utilising cURL for API integration.
A. Choosing the Right API
The first step is identifying an appropriate API that aligns with your project’s requirements. Popular options for WordPress development include:
- Payment Gateways: Integrate secure online payment functionalities like PayPal or Stripe.
- Social Media APIs: Allow users to share content directly on social media platforms.
- Marketing Automation Tools: Seamlessly connect your website with marketing automation tools like Mailchimp for email capture.
- Weather Services: Display real-time weather information on your website.
Once you’ve chosen the API, thoroughly review its documentation to understand its functionalities, supported request methods, and authentication requirements.
B. Setting Up the cURL Request
With the API selected, let’s configure the cURL request. Here are the key components:
- URL Construction: Build the API endpoint URL, including any dynamic parameters required for specific data retrieval.
- Authentication: Depending on the API, you might need to include an API key, access token, or other authentication credentials within your request header.
- Request Type: Specify the type of request (GET for retrieving data, POST for submitting data, PUT for updating data, etc.).
- Headers: Define the header information informing the server about the format of the expected response (e.g., application/json for JSON data).
Here’s an example of a basic cURL request structure in PHP:
PHP
$curl = curl_init('https://api.example.com/data'); // Replace with actual API endpoint URL
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true); // Set to capture the response
curl_setopt($curl, CURLOPT_HTTPHEADER, array('Content-Type: application/json')); // Set header for JSON data
// Add authentication details if required
$response = curl_exec($curl); // Execute the request
curl_close($curl); // Close the cURL connection
$data = json_decode($response); // Parse the JSON response
// Process the retrieved data
This is a simplified example. The specific code will vary depending on the chosen API and its requirements.
C. Processing the API Response
Once the request is executed, you’ll receive a response from the API server. This response typically contains data in a structured format like JSON or XML. Your code needs to handle and process this data effectively.
Here’s where your WordPress development skills come into play. You can leverage built-in functions like json_decode to parse JSON responses and extract the relevant information.
It’s crucial to consider potential error scenarios. The API might return an error code if the request is invalid or the data is unavailable. Implement proper error handling to gracefully handle such situations and provide informative messages to the user.
The following code snippets demonstrate practical cURL usage for different API integration scenarios. Remember to replace placeholders like API URLs and keys with your specific details.
1. Retrieving Data with a GET Request:
This snippet showcases fetching data from an API endpoint using a GET request:
PHP
$api_url = 'https://api.example.com/weather?city=london'; // Replace with actual API endpoint
$curl = curl_init($api_url);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($curl);
curl_close($curl);
$weather_data = json_decode($response);
// Access and process weather data from $weather_data object
if (isset($weather_data->cod) && $weather_data->cod != 200) {
echo 'Error: API request failed.';
} else {
// Display weather information on your website
}
2. Sending Data with a POST Request:
This snippet demonstrates sending data to an API using a POST request:
PHP
$api_url = 'https://api.example.com/users';
$data = array(
'name' => 'John Doe',
'email' => 'john.doe@example.com',
);
$curl = curl_init($api_url);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
curl_setopt($curl, CURLOPT_POST, true);
curl_setopt($curl, CURLOPT_POSTFIELDS, json_encode($data)); // Encode data as JSON
$response = curl_exec($curl);
curl_close($curl);
$response_data = json_decode($response);
// Process the API response based on success or failure
3. Authentication with an API Key:
This snippet shows including an API key in the request header for authentication:
PHP
$api_url = 'https://api.example.com/data';
$api_key = 'YOUR_API_KEY'; // Replace with your actual API key
$curl = curl_init($api_url);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
curl_setopt($curl, CURLOPT_HTTPHEADER, array('Authorization: Bearer ' . $api_key));
// ... rest of the code as before
These are just a few examples to illustrate the concept. The possibilities are vast, and the specific code will depend on the chosen API and its functionalities.
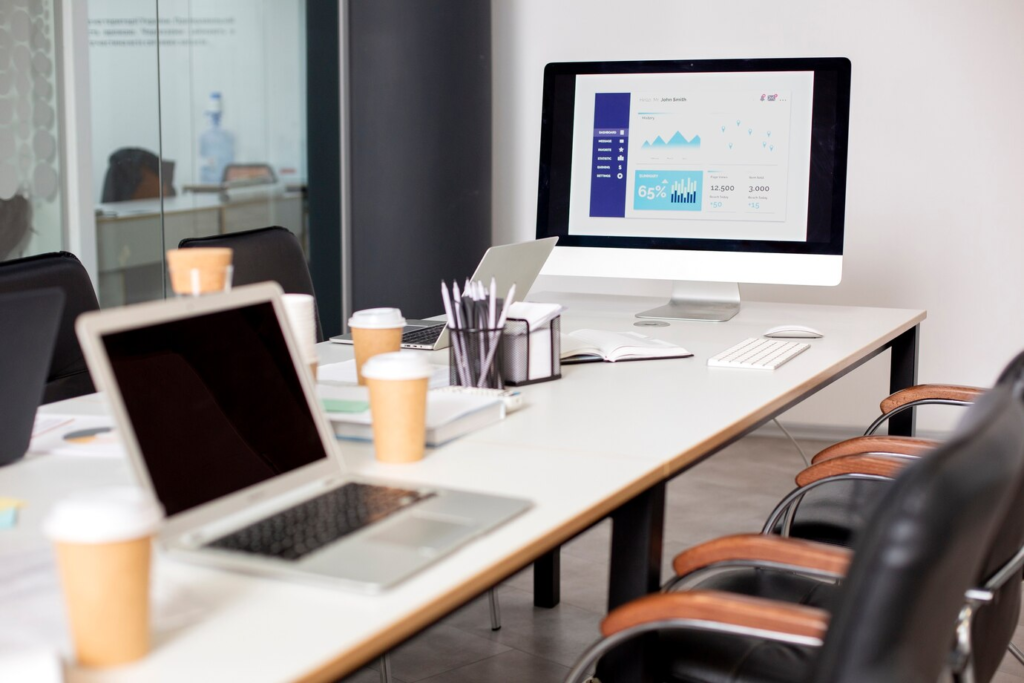
Security Considerations for cURL Integration
While cURL offers powerful functionalities, security is paramount. Here are some key considerations:
- Never store API keys directly in your code. Instead, use environment variables or a secure configuration file to manage sensitive information.
- Validate user input before sending it to an API. This helps prevent malicious code injection attempts.
- Sanitize data retrieved from the API. Ensure it’s safe for processing and display within your website.
- Consider using HTTPS for secure communication with the API server. This encrypts data transmission, protecting sensitive information.
By following these security best practices, you can minimise risks and ensure a secure API integration experience.
Conclusion
cURL unlocks a world of possibilities for integrating third-party APIs into your WordPress projects. It empowers you to connect your website with various external services and enhance user experience. By understanding the fundamentals of cURL and following the outlined steps, you can leverage its capabilities for seamless API integrations. Remember to choose the right API for your needs, configure requests appropriately, handle responses effectively, and prioritise security practices. With this knowledge, you can bridge the gap between your WordPress website and the vast ecosystem of third-party APIs, taking your development projects to the next level.